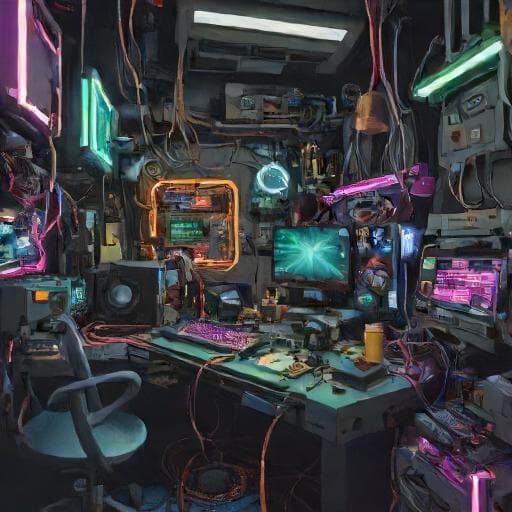
Loading...
Loading...
Javascript out of the box is a dynamic programming language, but does not provide great tooling and type safety like some typed languages. This is where typescript shines.
Typescript provides a type system to existing javascript. Our variable can now have a types and interfaces associated with them. Typescript also provides a great tooling system, for our IDEs, some of which are autocomplete, type annotations, etc.
One thing to keep in mind is that "all javascript is considered typescript but not all typescript is considered javascript".
This makes it very easy to migrate existion javascript projects to typescript, all we have to do is update the .js/.jsx file extension to .ts/.tsx and thats it.
With typescripts type annotations, we are able to spot bugs in our code sooner for example.
example: accessing undefined properties using JSconst me = {name: 'ziaul', hobby: 'code code code', location: 'NYC'}
console.log(me.age) // this code will run with out indicating any errors, it will just print undefined
the same code in typeScript below will produce the following
const me = {name: 'ziaul', hobby: 'code code code', location: 'NYC'}
console.log(me.someRandomProperty) // this code will indicate an error in the IDE
Property 'someRandomProperty' does not exist on type 'name: string; hobby: string; location: string;'
As you can see Typescript helps us identify the issue before we run the code.
Typescript also comes with great support for autocomplete in our IDE environments.
Take a look at this js example below
you can see that it doesnot really provide very good autocomplete, however the same code using Typescript will yeild better autocomplete